How to Give Functionality to a Button With Javascript
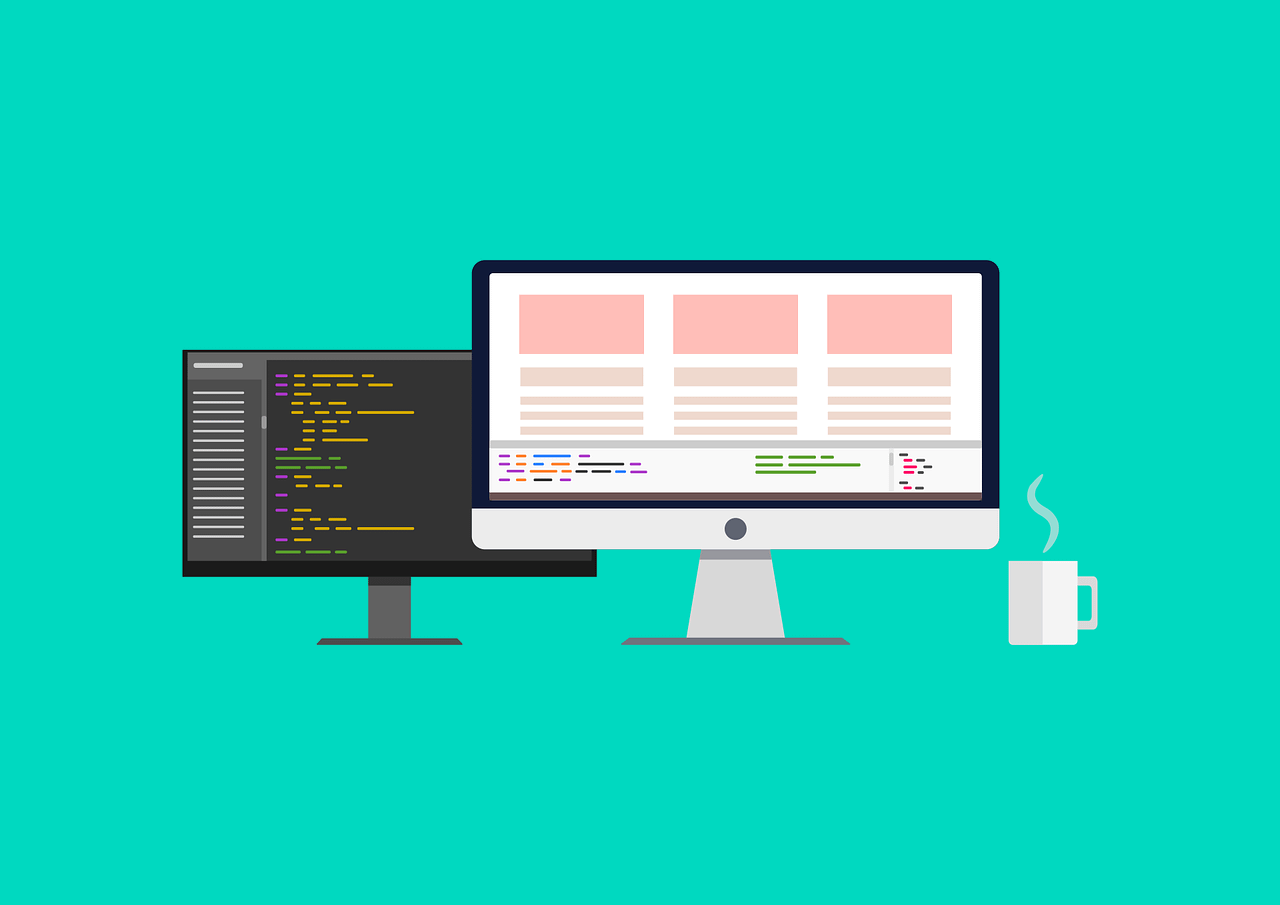
Javascript gives us the possibility to accessing what we call the DOM, which basically always happens because everything we do in javascript directly or indirectly infers on the HTML or CSS of the site, so there is no mystery in it. And a common way to do it is through what is known as events. And by this, we mean that when an “action occurs” on our website, we can trigger a code sequence. These events can be many, but we are going to focus on the ones that are usually used in buttons, which are onclick, onmouseover and onmouseout.
The same operating logic applies to all of them, so it is enough to explain the most important one (onclick), and the rest can be deduced without much effort.
We are going to do it in just three steps:
First. You must make a button in HTML making sure to give it an id.
<form>
<input type=“button“ id=“myButton“ value=“give me a click!“/>
</form>
Second. Create a variable and assign everything that is obtained from that identifier. The complete sentence says: “we are going to access the document obtaining the id (myButton) and those values will be captured in the variable (testButton)”.
<script>
var testButton = document.getElementById(“myButton“);
Third. Remember that the variable “testButton” has captured the data of the id. We access that variable and with “addEventListener” we will be attentive to when an action is executed with the button, in this case, the “click” action. Then we add a function, this can be anonymous, it does not need a name, and between its curly brackets, we put the action. In this case, it is just a call to the console, but any function you need could be executed here.
testButton.addEventListener(‘click‘,function(){
console.log(‘You gave me a click, Well done!‘);
})
</script>
And we finished. That is all the code that is required.
There is a second alternative and it comes from the very essence of Object-Oriented Programming.
Look at the code:
<form>
<input type=“button“ id=“myButton” value=“give me a click!“/>
</form>
<script>
var testButton = document.getElementById(“myButton“);
testButton.onclick = function(){
console.log(‘You gave me a click, Well done! again‘);
}
</script>
As you can see, it’s almost the same code, it continues to use a variable to capture the information of the button by means of its id, and the same anonymous function that executes the call to the console. The difference is in a single line: we access the button property, “onclick”, and that way we avoid using the event.
Interesting!
—————-
We'd love to know your opinion. Leave us a message or follow us! we are on social networks
We have more articles and tutorials that may be of interest to you.
0 Comments